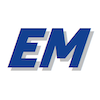 |
EM-ODP
3.7.0
Event Machine on ODP
|
Go to the documentation of this file.
31 #ifndef EVENT_MACHINE_HOOKS_H_
32 #define EVENT_MACHINE_HOOKS_H_
34 #pragma GCC visibility push(default)
114 int num_act,
int num_req, uint32_t size,
160 em_event_group_t event_group);
463 #pragma GCC visibility pop
em_api_hook_alloc_t alloc_hook
em_api_hook_send_t send_hook
em_api_hook_free_t free_hook
em_status_t em_hooks_unregister_alloc(em_api_hook_alloc_t func)
em_idle_hook_to_idle_t to_idle_hook
em_idle_hook_while_idle_t while_idle_hook
void(* em_idle_hook_to_active_t)(void)
void(* em_api_hook_send_t)(const em_event_t events[], int num, em_queue_t queue, em_event_group_t event_group)
void(* em_idle_hook_while_idle_t)(void)
void(* em_idle_hook_to_idle_t)(uint64_t to_idle_delay_ns)
em_status_t em_hooks_unregister_to_idle(em_idle_hook_to_idle_t func)
em_status_t em_hooks_register_free(em_api_hook_free_t func)
em_status_t em_hooks_register_alloc(em_api_hook_alloc_t func)
em_status_t em_hooks_unregister_send(em_api_hook_send_t func)
em_status_t em_hooks_unregister_to_active(em_idle_hook_to_active_t func)
em_status_t em_hooks_register_while_idle(em_idle_hook_while_idle_t func)
em_idle_hook_to_active_t to_active_hook
void(* em_api_hook_alloc_t)(const em_event_t events[], int num_act, int num_req, uint32_t size, em_event_type_t type, em_pool_t pool)
em_status_t em_hooks_unregister_free(em_api_hook_free_t func)
em_status_t em_hooks_register_send(em_api_hook_send_t func)
em_status_t em_hooks_unregister_while_idle(em_idle_hook_while_idle_t func)
void(* em_api_hook_free_t)(const em_event_t events[], int num)
em_status_t em_hooks_register_to_active(em_idle_hook_to_active_t func)
em_status_t em_hooks_register_to_idle(em_idle_hook_to_idle_t func)