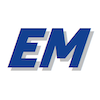 |
EM-ODP
3.7.0
Event Machine on ODP
|
Go to the documentation of this file.
31 #ifndef EVENT_MACHINE_DISPATCHER_H_
32 #define EVENT_MACHINE_DISPATCHER_H_
34 #pragma GCC visibility push(default)
98 EM_DISPATCH_DURATION_LAST
534 em_event_t events[],
int num,
535 em_queue_t *queue,
void **q_ctx);
654 #pragma GCC visibility pop
em_dispatch_duration_select_t
EM dispatch duration selection flags.
void(* em_dispatch_exit_func_t)(em_eo_t eo)
em_dispatch_duration_select_t select
uint64_t em_dispatch(uint64_t rounds)
em_status_t em_dispatch_rounds(uint64_t rounds, const em_dispatch_opt_t *opt, em_dispatch_results_t *results)
Run the EM dispatcher for a given number of dispatch-rounds.
@ EM_DISPATCH_DURATION_NS
em_status_t em_dispatch_ns(uint64_t ns, const em_dispatch_opt_t *opt, em_dispatch_results_t *results)
Run the EM dispatcher for a given amount of time (in nanoseconds).
em_status_t em_dispatch_register_enter_cb(em_dispatch_enter_func_t func)
@ EM_DISPATCH_DURATION_FOREVER
em_status_t em_dispatch_duration(const em_dispatch_duration_t *duration, const em_dispatch_opt_t *opt, em_dispatch_results_t *results)
Run the EM dispatcher for a certain duration with options.
@ EM_DISPATCH_DURATION_EVENTS
uint32_t __internal_check
@ EM_DISPATCH_DURATION_NO_EVENTS_ROUNDS
void em_dispatch_opt_init(em_dispatch_opt_t *opt)
Initialize the EM dispatch options.
@ EM_DISPATCH_DURATION_ROUNDS
void(* em_dispatch_enter_func_t)(em_eo_t eo, void **eo_ctx, em_event_t events[], int num, em_queue_t *queue, void **q_ctx)
em_status_t em_dispatch_unregister_enter_cb(em_dispatch_enter_func_t func)
em_status_t em_dispatch_unregister_exit_cb(em_dispatch_exit_func_t func)
em_status_t em_dispatch_events(uint64_t events, const em_dispatch_opt_t *opt, em_dispatch_results_t *results)
Run the EM dispatcher until a given number of events have been dispatched.
em_status_t em_dispatch_register_exit_cb(em_dispatch_exit_func_t func)
@ EM_DISPATCH_DURATION_NO_EVENTS_NS