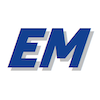 |
EM-ODP
3.7.0
Event Machine on ODP
|
Go to the documentation of this file.
31 #ifndef EVENT_MACHINE_PACKET_H_
32 #define EVENT_MACHINE_PACKET_H_
34 #pragma GCC visibility push(default)
326 #pragma GCC visibility pop
void * em_packet_push_tail(em_event_t pktev, uint32_t len)
Push out the end of the packet into the tailroom.
void * em_packet_pointer(em_event_t pktev)
em_status_t em_packet_reset(em_event_t pktev, uint32_t size)
uint32_t em_packet_headroom(em_event_t pktev)
Packet event headroom length.
void * em_packet_pull_tail(em_event_t pktev, uint32_t len)
void * em_packet_push_head(em_event_t pktev, uint32_t len)
Push out the beginning of the packet into the headroom.
void * em_packet_resize(em_event_t pktev, uint32_t size)
Resize a packet event.
uint32_t em_packet_tailroom(em_event_t pktev)
Packet event tailroom length.
void * em_packet_pointer_and_size(em_event_t pktev, uint32_t *size)
Get the packet event data pointer as well as the packet size.
void * em_packet_pull_head(em_event_t pktev, uint32_t len)
Pull in the beginning of the packet from the headroom.
uint32_t em_packet_size(em_event_t pktev)
Get the packet size.